Dec 03, 2024 | 414 words | 4 min read
6.3.2. Task 2#
Learning Objectives#
Create and implement user-defined functions in Python; Construct logic
operations using comparison, membership, and logical operators to generate control flow
statements in Python; Utilize conditional
if
-elif
-else
statements while programming in
Python, as well as previously learned objectives such as: Output data from a
function to the screen in Python; Apply course code standard in development
of Python scripts; Modularize and comment code in Python for
readability and reusability.
Introduction#
Good water volume estimates are necessary for safe swimming pool maintenance. A swimming pool manufacturer has asked you to develop a pool volume calculator for their customers. You will create one main function and a set of sub-functions to calculate and display the volume of 3 swimming pool shapes offered by the pool manufacturer.
The manufacturer offers three pool shapes:
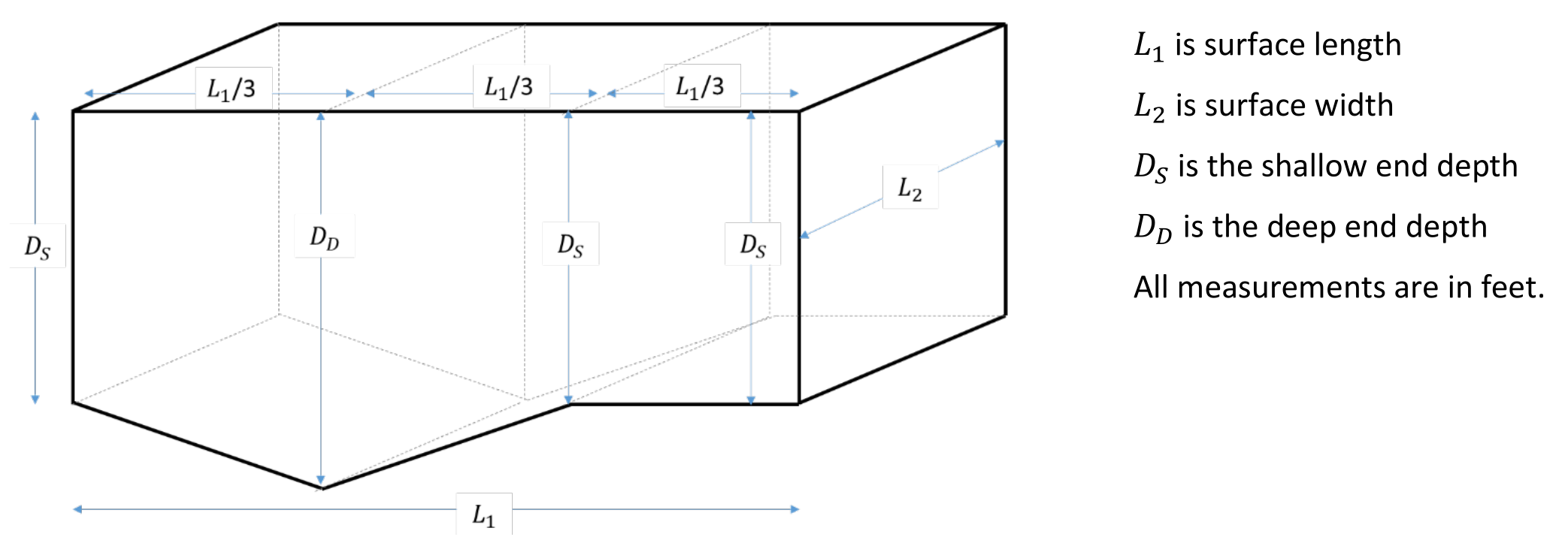
Fig. 6.2 The standard pool.#
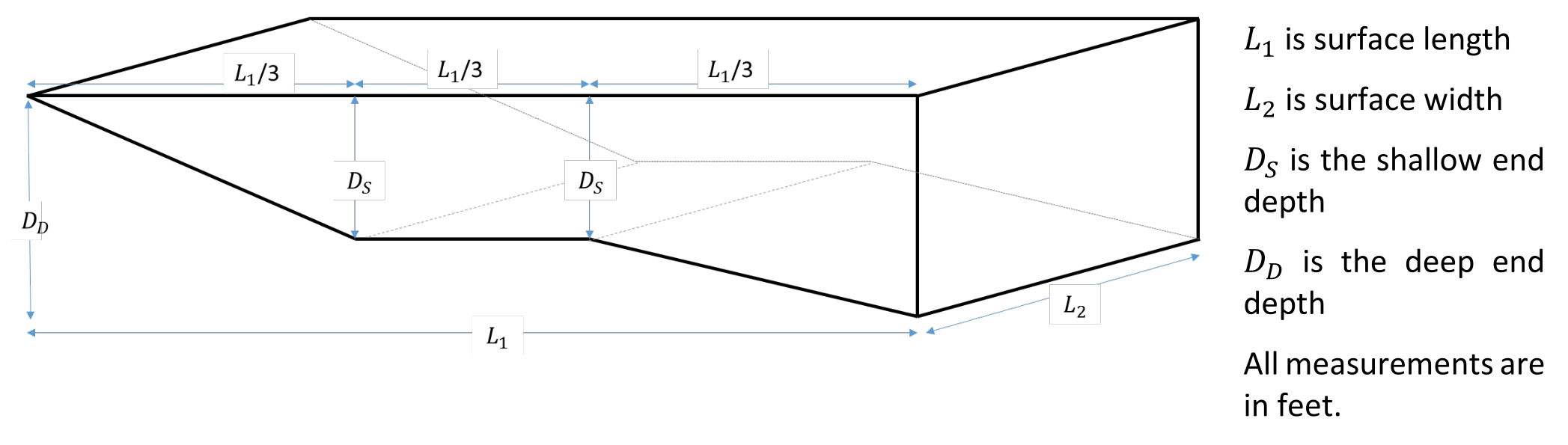
Fig. 6.3 The ramp pool.#
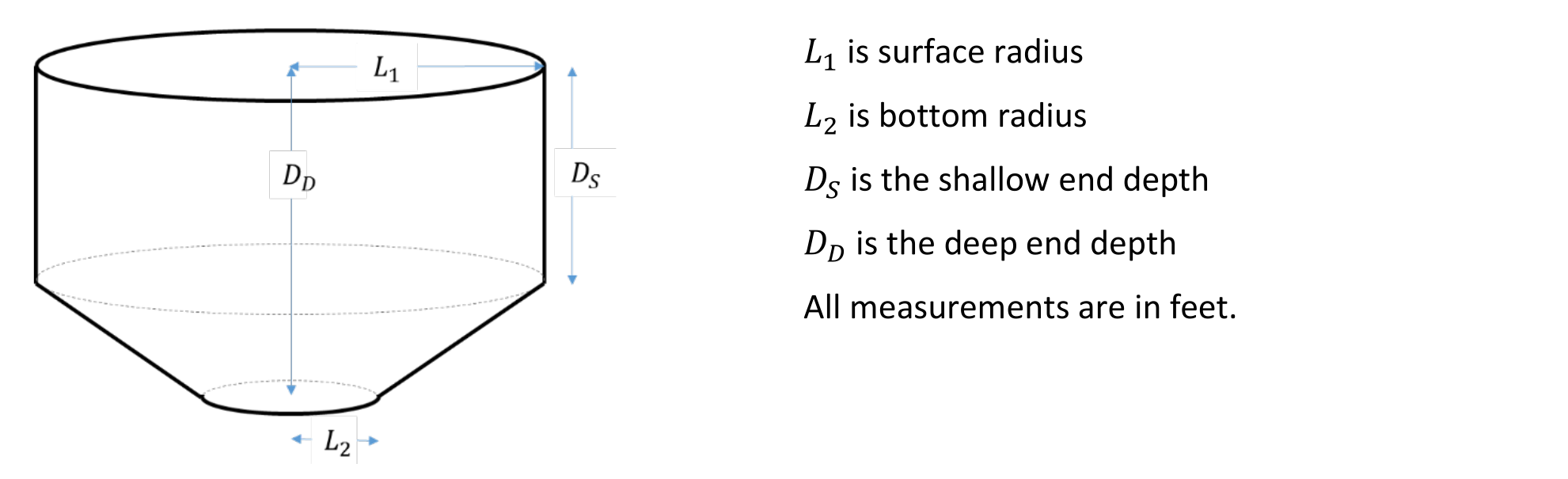
Fig. 6.4 The round pool.#
Task Instructions#
Write one function for each pool type shown above in a file named
py2_ind_2_functions_username.py. The
functions must be named standard
, ramp
, and round
respectively. Each function must meet the following requirements:
Accept \(L_1\), \(L_2\), \(D_s\), and \(D_d\) as scalar arguments. The arguments must be in this order, and their values will be in feet.
Display a meaningful error message and return
None
if a negative number is passed in as an input argument.Return 1 scalar output: the pool volume in gallons. In the case of invalid input, the return value should be
None
.Conversion rate from cubic ft to gallons: 1 cubic ft = 7.48 gallons
Follow good programming standards.
You will then write your main
function in a separate file named
py2_ind_2_main_username.py that calls the
proper function from the functions file to display the pool volume to the terminal. Your
main
function should do the following:
Prompt the user for the name of the pool (one of Standard, Ramp, or Round).
Display a meaningful error message and stop if the input pool name does not match the available pool shapes.
Prompt the user to enter the four dimensions of the pool.
Display the pool name and pool volume in gallons for only the pool requested.
Follow good programming standards.
Sample Output#
All measurements are in feet. Use the values in Table 6.9 below to test your program.
Case |
Name |
length/radius |
width/radius |
shallow end depth |
deep end depth |
---|---|---|---|---|---|
1 |
Standard |
20 |
10 |
5 |
10 |
2 |
Ramp |
20 |
10 |
4 |
8 |
3 |
Round |
20 |
10 |
3 |
12 |
4 |
Standard |
20 |
10 |
5 |
-10 |
5 |
tsandard |
Ensure your program’s output matches the provided samples exactly. This includes all characters, white space, and punctuation. In the samples, user input is highlighted like this for clarity, but your program should not highlight user input in this way.
Case 1 Sample Output
$ python3 py2_ind_2_main_username.py Enter the name of the pool to calculate (Standard, Ramp, or Round): Standard Enter the surface length or radius. 20 Enter the surface width or bottom radius. 10 Enter the shallow end depth. 5 Enter the deep end depth. 10 The volume of the Standard pool with your dimensions is 9,973.33 gallons.
Case 2 Sample Output
$ python3 py2_ind_2_main_username.py Enter the name of the pool to calculate (Standard, Ramp, or Round): Ramp Enter the surface length or radius. 20 Enter the surface width or bottom radius. 10 Enter the shallow end depth. 4 Enter the deep end depth. 8 The volume of the Ramp pool with your dimensions is 5,984.00 gallons.
Case 3 Sample Output
$ python3 py2_ind_2_main_username.py Enter the name of the pool to calculate (Standard, Ramp, or Round): Round Enter the surface length or radius. 20 Enter the surface width or bottom radius. 10 Enter the shallow end depth. 3 Enter the deep end depth. 12 The volume of the Round pool with your dimensions is 77,547.07 gallons.
Case 4 Sample Output
$ python3 py2_ind_2_main_username.py Enter the name of the pool to calculate (Standard, Ramp, or Round): Standard Enter the surface length or radius. 20 Enter the surface width or bottom radius. 10 Enter the shallow end depth. 5 Enter the deep end depth. -10 Please enter valid dimensions.
Case 5 Sample Output
$ python3 py2_ind_2_main_username.py Enter the name of the pool to calculate (Standard, Ramp, or Round): tsandard Please run the program again and enter a valid pool name.