Dec 03, 2024 | 541 words | 5 min read
10.2.3. Task 3#
Learning Objectives#
Encode a binary message into an image by altering the least significant bits of the pixel values.
Task Instructions#
Save the flowcharts for each of your tasks in tp2_team_teamnumber.pdf You will also need to include these flowcharts in your final report.
Create a function that takes the binary message, an input image file path, and an output image file path as its only arguments. This function should first load the input image and check if the binary message can fit into it. If the binary message is too long to be encoded into the image, the function should print an error message. Otherwise, the function should encode the binary message into the image by altering the least significant bits of the pixel values. Start in the upper left corner of the image, and proceed left to right across each row. For color images, iterate over each channel (R, G, B) of each pixel before moving to the next pixel. Write the modified image to the output file path.
Finally, create a main
function in your Python program that builds
on the code you developed in Task 2 and uses the function described
above to encode a message into an image. Your main
function should prompt the
user to enter a plaintext message, a key, a start sequence, an end sequence, a path to
an image file, and a path to an output file. It should then encrypt the message using
the Vigenère cipher, generate the binary message, encode the binary message into the
image, and save the modified image to the output file. Finally, it should display a
message indicating whether the message has been successfully encoded into the image and
saved to the specified path. Use the files provided in the
Table 10.35 to test your code.
Note
The matplotlib
module converts grayscale images to color images when they are
saved using imsave
. So you will need to use the PIL
library to when
saving your output images. You can use the save
method of the Image
class to save the image to a file. Here is an example of how you can save a grayscale
image using the PIL
library:
from PIL import Image
pil_image = Image.fromarray(img_array, mode="L")
pil_image.save(img_path)
For color images, set the mode argument to RGB
.
See the PIL documentation for more information.
Save your program as tp2_team_3_teamnumber.py.
Notes
The encoded image should appear visually identical to the original image, as changes to the LSBs are typically imperceptible to the human eye.
The encoded message will not be visible when viewing the image, but it can be extracted programmatically by decoding the LSBs.
The program should handle both grayscale and color images effectively, ensuring that the image data is properly prepared for encoding.
Image File Name |
Description |
---|---|
Original grayscale image |
|
grayscale image with Vigenère encrypted message encoded |
|
Original color image |
|
Color mage with Vigenère encrypted message encoded |
Sample Output#
Use the values in Table 10.36 below to test your program.
Case |
plaintext |
Vigenère key |
start_seq |
end_seq |
input image path |
output image path |
---|---|---|---|---|---|---|
1 |
hello |
Beau |
007 |
700 |
ref_col.png |
ref_col_v.png |
2 |
World |
Bear |
007 |
700 |
ref_gry.png |
ref_gry_v.png |
3 |
Hello World |
Bear |
007 |
700 |
ref_gry.png |
error.png |
Ensure your program’s output matches the provided samples exactly. This includes all characters, white space, and punctuation. In the samples, user input is highlighted like this for clarity, but your program should not highlight user input in this way.
Case 1 Sample Output
$ python3 tp2_team_3_teamnumber.py Enter the plaintext you want to encrypt: hello Enter the key for Vigenere cipher: Beau Enter the start sequence: 007 Enter the end sequence: 700 Enter the path of the image: ref_col.png Enter the path for the encoded image: ref_col_v.png Encrypted Message using Vigenere Cipher: iilfp Binary output message: 00110000 00110000 00110111 01101001 01101001 01101100 01100110 01110000 00110111 00110000 00110000 Message successfully encoded and saved to: ref_col_v.png
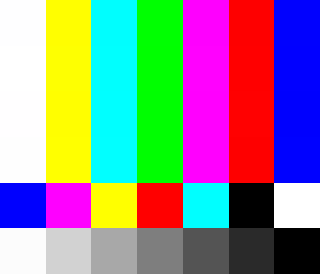
Fig. 10.4 Case_1_ref_col_v.png#
Case 2 Sample Output
$ python3 tp2_team_3_teamnumber.py Enter the plaintext you want to encrypt: World Enter the key for Vigenere cipher: Bear Enter the start sequence: 007 Enter the end sequence: 700 Enter the path of the image: ref_gry.png Enter the path for the encoded image: ref_gry_v.png Encrypted Message using Vigenere Cipher: Xsrce Binary output message: 00110000 00110000 00110111 01011000 01110011 01110010 01100011 01100101 00110111 00110000 00110000 Message successfully encoded and saved to: ref_gry_v.png
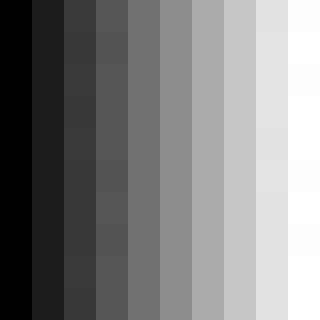
Fig. 10.5 Case_2_ref_gry_v.png#
Case 3 Sample Output
$ python3 tp2_team_3_teamnumber.py Enter the plaintext you want to encrypt: Hello World Enter the key for Vigenere cipher: Bear Enter the start sequence: 007 Enter the end sequence: 700 Enter the path of the image: ref_gry.png Enter the path for the encoded image: error.png Given message is too long to be encoded in the image. Encrypted Message using Vigenere Cipher: Iilcp Wfspd Binary output message: 00110000 00110000 00110111 01001001 01101001 01101100 01100011 01110000 00100000 01010111 01100110 01110011 01110000 01100100 00110111 00110000 00110000